画像を拡縮する際の処理を確認していきます。
resized_image = cv2.resize(image, (width * 2, height * 2), interpolation=cv2.INTER_LINEAR)
また、以下のように書くこともできます。
resized_image = cv2.resize(image, None, fx=0.5, fy=0.5, interpolation=cv2.INTER_NEAREST)
補間方法(interpolation)は下記の通りです。
- INTER_NEAREST – 最近傍補間
- INTER_LINEAR – バイリニア補間(デフォルト)
- INTER_AREA – ピクセル領域の関係を利用したリサンプリング。画像を大幅に縮小する場合、モアレを避けることができる手法。画像を拡大する場合は、INTER_NEARESTと同様になる
- INTER_CUBIC – 4×4 の近傍領域を利用するバイキュービック補間
- INTER_LANCZOS4 – 8×8 の近傍領域を利用するLanczos法での補間
サンプルコード
import cv2
import numpy as np
import matplotlib.pyplot as plt
image = cv2.imread("Lenna.png")
image = cv2.cvtColor(image, cv2.COLOR_BGR2RGB)
image = cv2.resize(image, None, fx=0.1, fy=0.1,
interpolation=cv2.INTER_NEAREST)
h, w = image.shape[:2]
plt.subplot(2, 3, 1)
plt.title('original')
plt.imshow(image)
plt.subplot(2, 3, 2)
resized_image = cv2.resize(image, (w * 2, h * 2),
interpolation=cv2.INTER_NEAREST)
plt.title('cv2.INTER_NEAREST')
plt.imshow(resized_image)
plt.subplot(2, 3, 3)
resized_image = cv2.resize(image, (w * 2, h * 2),
interpolation=cv2.INTER_LINEAR)
plt.title('cv2.INTER_LINEAR')
plt.imshow(resized_image)
plt.subplot(2, 3, 4)
resized_image = cv2.resize(image, (w * 2, h * 2),
interpolation=cv2.INTER_AREA)
plt.title('cv2.INTER_AREA')
plt.imshow(resized_image)
plt.subplot(2, 3, 5)
resized_image = cv2.resize(image, (w * 2, h * 2),
interpolation=cv2.INTER_CUBIC)
plt.title('cv2.INTER_CUBIC')
plt.imshow(resized_image)
plt.subplot(2, 3, 6)
resized_image = cv2.resize(image, (w * 2, h * 2),
interpolation=cv2.INTER_LANCZOS4)
plt.title('cv2.INTER_LANCZOS4')
plt.imshow(resized_image)
plt.show()
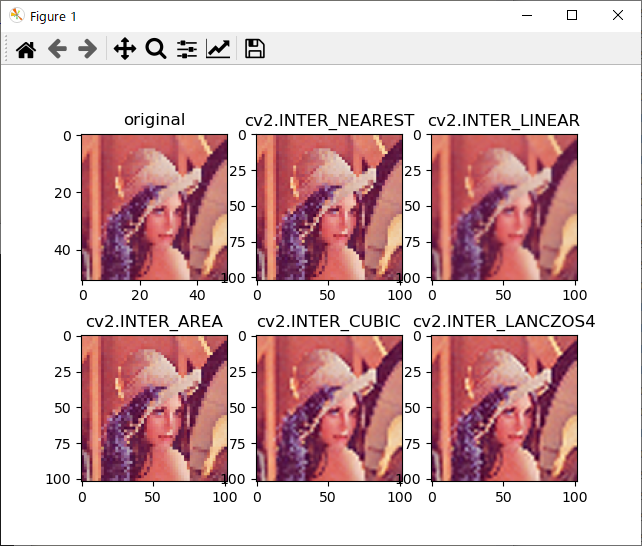